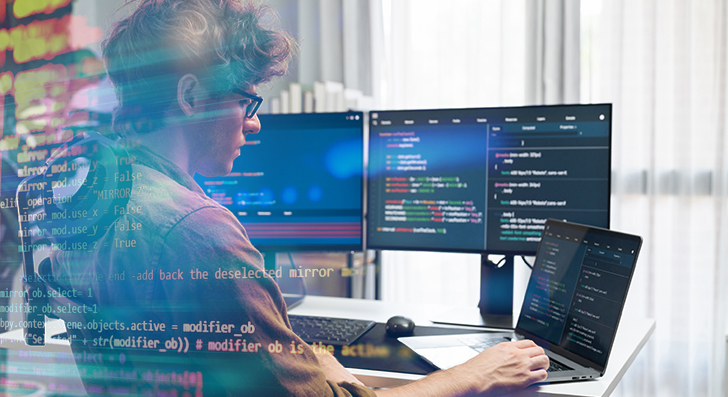
Scalability suggests your software can tackle expansion—a lot more customers, more details, and much more traffic—without the need of breaking. For a developer, creating with scalability in mind will save time and tension afterwards. Listed here’s a transparent and practical tutorial that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be element of your prepare from the beginning. A lot of applications fall short when they increase fast because the initial structure can’t take care of the additional load. As being a developer, you'll want to Believe early regarding how your system will behave under pressure.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. As an alternative, use modular style and design or microservices. These styles split your application into smaller, independent areas. Each individual module or services can scale on its own devoid of influencing The full process.
Also, consider your database from day just one. Will it have to have to handle a million end users or merely a hundred? Choose the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Yet another critical position is to stop hardcoding assumptions. Don’t generate code that only is effective under current circumstances. Take into consideration what would take place In the event your person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use layout designs that help scaling, like information queues or event-pushed programs. These support your app manage a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you're not just preparing for fulfillment—you might be cutting down long run complications. A properly-planned program is easier to maintain, adapt, and develop. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the right databases is actually a important Section of creating scalable applications. Not all databases are crafted the exact same, and using the Incorrect you can sluggish you down or even induce failures as your app grows.
Start out by knowledge your information. Can it be hugely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely potent with associations, transactions, and consistency. Additionally they support scaling approaches like study replicas, indexing, and partitioning to take care of a lot more targeted visitors and knowledge.
If your details is much more adaptable—like user action logs, item catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured details and may scale horizontally additional easily.
Also, take into account your read and compose styles. Are you undertaking many reads with fewer writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases which can handle large produce throughput, or simply event-primarily based details storage methods like Apache Kafka (for short term knowledge streams).
It’s also good to think ahead. You may not want Innovative scaling capabilities now, but deciding on a database that supports them implies you gained’t need to have to change later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data depending on your access patterns. And constantly keep an eye on databases effectiveness while you improve.
Briefly, the appropriate databases will depend on your application’s construction, pace requires, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll preserve plenty of difficulty later.
Improve Code and Queries
Speedy code is vital to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all unnecessary. Don’t pick the most intricate Answer if a straightforward just one is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling resources to uncover bottlenecks—spots exactly where your code usually takes far too very long to run or takes advantage of an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every question only asks for the information you truly want. Stay clear of Pick *, which fetches everything, and alternatively find certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically across massive tables.
If you recognize a similar information currently being asked for repeatedly, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t should repeat expensive operations.
Also, batch your database functions after you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to take a look at with large datasets. Code and queries that perform wonderful with a hundred documents could possibly crash when they have to handle 1 million.
In a nutshell, scalable apps are rapidly applications. Maintain your code restricted, your queries lean, and use caching when needed. These actions assist your application remain easy and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more customers and even more site visitors. If almost everything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment aid keep your app fast, secure, and scalable.
Load balancing spreads incoming website traffic throughout many servers. In place of just one server undertaking each of the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it could be reused swiftly. When customers ask for precisely the same info all over again—like an item web page or perhaps a profile—you don’t really need to fetch it with the database when. It is possible to serve it with the cache.
There are two prevalent varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And constantly make sure your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but impressive resources. Jointly, they help your app take care of extra end users, continue to be quick, and Get well from issues. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To build scalable programs, you require applications that let your app expand quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you are able to include additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability applications. You could deal with setting up your application as an alternative to controlling infrastructure.
Containers are Yet another critical Resource. A container deals your app and everything it needs to operate—code, libraries, options—into a single unit. This can make it uncomplicated to move your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the most popular Software for this.
Once your app utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into solutions. You could update or scale elements independently, which can be perfect for functionality and reliability.
Briefly, making use of cloud and container applications implies you can scale rapidly, deploy effortlessly, and Get well rapidly when challenges occur. If you prefer your app to improve with out boundaries, start employing these resources early. They help save time, reduce chance, and help you remain centered on building, not fixing.
Watch Everything
In case you don’t observe your application, you gained’t know when factors go Completely wrong. Monitoring aids the thing is how your application is carrying out, place difficulties early, and make much better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just keep track of your servers—check your app way too. Regulate how long it takes for customers to load webpages, how often mistakes take place, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring inside your code.
Create alerts for crucial troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This helps you resolve problems quick, often before buyers even detect.
Checking is additionally helpful when you make variations. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic hurt.
As your app grows, targeted visitors and knowledge boost. Without having monitoring, you’ll miss out on signs of website hassle right up until it’s as well late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring helps you maintain your app reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for major organizations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the correct instruments, you are able to Create applications that expand effortlessly with out breaking stressed. Get started little, Assume big, and Construct good.